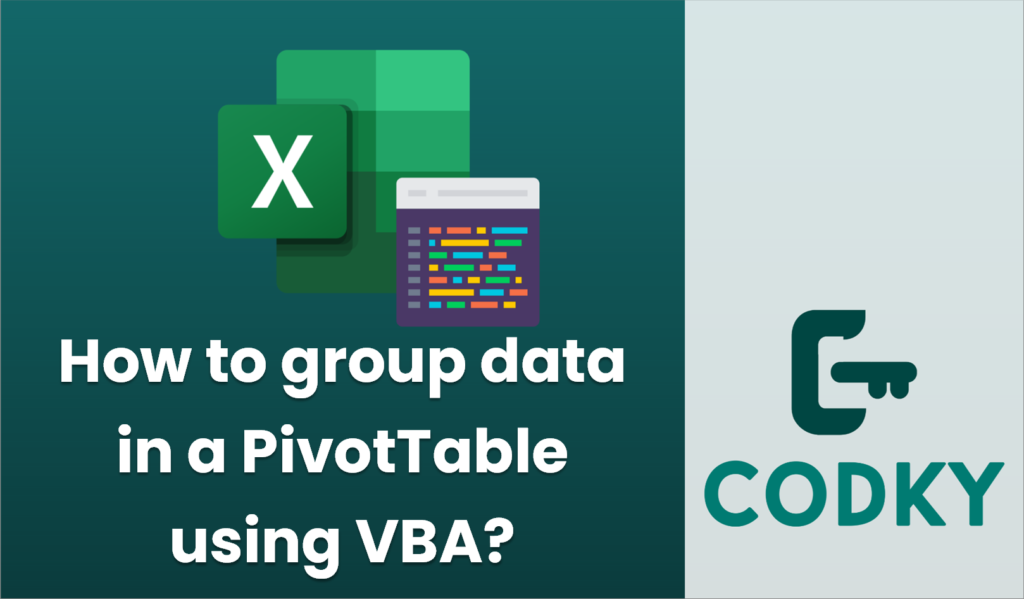
Grouping data in a PivotTable using VBA can be a useful way to summarize and analyze data in Excel. Here’s a basic guide on how to do this:
Sub GroupPivotTableData()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
' Change this to your worksheet and PivotTable names
Set ws = ThisWorkbook.Sheets("Sheet1")
Set pt = ws.PivotTables("PivotTable1")
' Ensure the PivotTable is refreshed
pt.RefreshTable
' Change "Field1" to the name of the field you want to group
Set pf = pt.PivotFields("Field1")
' Check if the field is already grouped and ungroup if needed
On Error Resume Next
pf.ClearAllFilters
pf.Ungroup
On Error GoTo 0
' Example: Grouping numeric data in range of 10
pf.Group Start:=10, End:=100, By:=10
' Example: Grouping date data by month
'pf.Group Start:=DateValue("1/1/2020"), End:=DateValue("12/31/2020"), By:=1, _
Periods:=Array(False, False, False, True, False, False, False)
End Sub
- Open the Visual Basic for Applications Editor:
- Press `ALT` + `F11` to open the VBA editor in Excel.
- Insert a Module:
- In the VBA editor, go to `Insert` > `Module` to create a new module where you will write your VBA code.
- Write the VBA Code:
- Below is a sample VBA code to group data in a PivotTable:
- Customize the Code:
- Replace `”Sheet1″` and `”PivotTable1″` with the actual sheet name and PivotTable name.
- Replace `”Field1″` with the name of the field you want to group.
- Adjust the `Start`, `End`, `By`, and `Periods` parameters to suit your data.
- Run the Macro:
- Close the VBA editor and return to Excel.
- Press `ALT` + `F8`, select `GroupPivotTableData`, and click `Run`.
Additional Tips:
- Numeric Grouping: For numeric fields, you can specify the `Start`, `End`, and `By` parameters for range grouping.
- Date Grouping: For date fields, you can specify start and end dates and decide how to group (by days, months, quarters, etc.) using the `Periods` array.
- Error Handling: The use of `On Error Resume Next` helps prevent runtime errors if the field is not grouped. Adjust error handling based on your needs.
This script provides a structured approach to grouping fields in a PivotTable using VBA macros, which can be tailored to fit your particular dataset and analysis requirements.