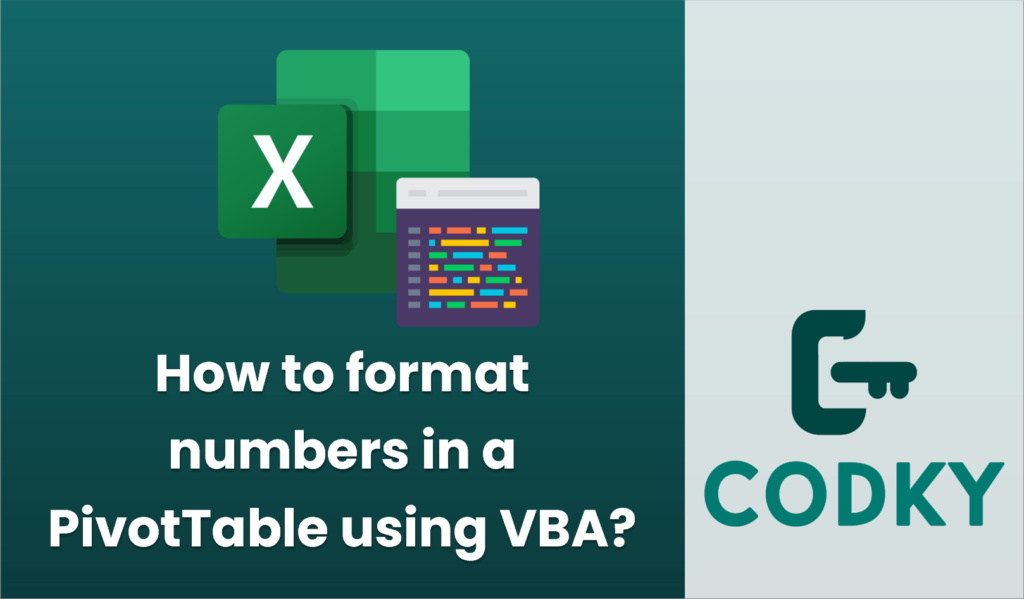
To format numbers in a PivotTable using VBA, you’ll need to work with the `PivotFields` and their associated properties. Here’s a step-by-step guide to help you do this:
- Access the PivotTable: First, ensure you have a reference to the PivotTable you want to format. This is often accessible through the worksheet where it’s located.
- Identify the PivotField: Determine which field(s) you want to format. This could be in the data area, row labels, or column labels.
- Apply Number Format: Set the `NumberFormat` property of the desired `PivotField` to the format string you need.
Here’s a basic example of how you could format numbers in a PivotTable using VBA:
Sub FormatPivotTableNumbers()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
' Assuming your PivotTable is on Sheet1
Set ws = ThisWorkbook.Sheets("Sheet1")
' Reference the first PivotTable on the sheet (change as necessary)
Set pt = ws.PivotTables(1)
' Format the data fields
For Each pf In pt.DataFields
' Change "0.00" to your desired number format
pf.NumberFormat = "0.00"
Next pf
' Optional: Format specific fields, e.g., a row field or a column field
' If you want to format a specific field, use its name
' Set pf = pt.PivotFields("YourFieldName")
' pf.NumberFormat = "0.00"
End Sub
Key Points:
- Worksheet and PivotTable Reference: Make sure you reference the correct worksheet and PivotTable. If your PivotTable is not the first one on the sheet, you might need to specify its index or explicitly name it.
- Number Format Strings: The `NumberFormat` property accepts a format string like “0.00”, “#,##0.00”, or even currency formats like “$#,##0.00”. Customize this string based on how you want numbers to appear.
- Specific Fields: If you need to format a specific field rather than all data fields, access it by its name using `pt.PivotFields(“FieldName”)`.
- PivotField Types: Remember that not all fields may need formatting. Typically, you apply number formats to data fields, but you might occasionally need to format row or column labels.
By using these steps, you should be able to effectively format your PivotTable numbers through VBA. Adjust the code as needed for your specific scenario and data layout.