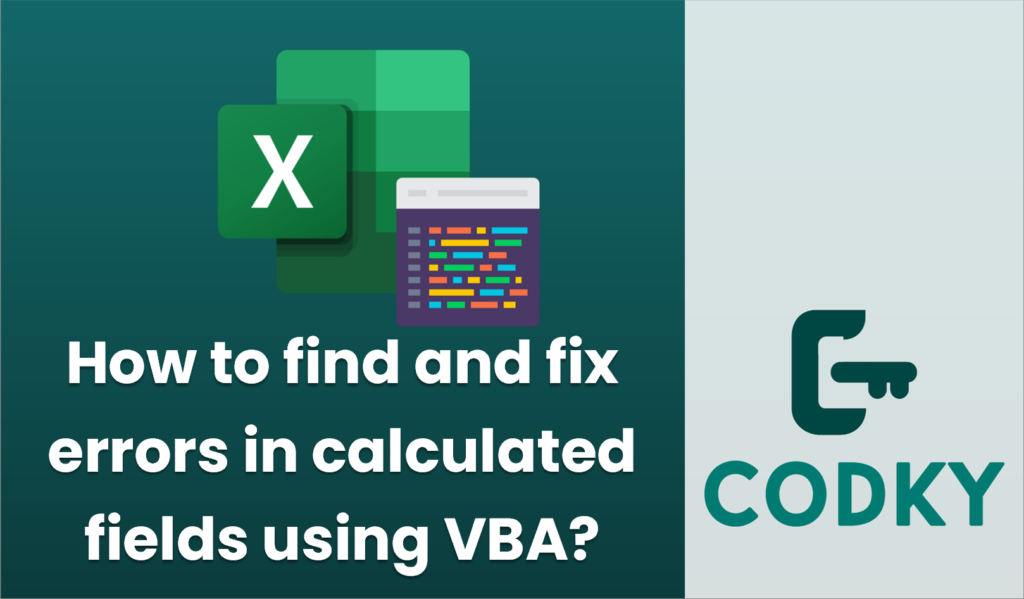
Finding and fixing errors in calculated fields using VBA can be an intricate process, but it’s manageable with the right steps. Here’s a general approach you can follow to troubleshoot and correct these errors:
Identify the Problem
- Understand the Calculated Field:
- Make sure you understand what the calculated field is supposed to do. This includes the logic, the source data, and the expected results.
- Review the Formula:
- Carefully review the formula or logic used in the calculated field. Look for syntax errors or logical mistakes.
- Recreate the Error:
- Try to reproduce the error consistently. Understand when and how it occurs. This will help in isolating the issue.
- Check Data Types:
- Ensure that the data types of the input fields match those expected by the calculation. Mismatch in data types can lead to errors.
Using VBA to Debug
On Error GoTo ErrorHandler
' Your VBA code here
Exit Sub
ErrorHandler:
MsgBox "Error " & Err.Number & ": " & Err.Description, vbExclamation
- Enable Error Handling:
- Start your VBA code with proper error handling to catch and display detailed error messages. Example:
- Log Details:
- Implement logging to understand where the error occurs. This could involve writing to a debug window or a text file.
- Step Through the Code:
- Use the VBA editor to step through your code. Use breakpoints to stop execution at crucial points and inspect variables.
- Immediate Window:
- Use the Immediate Window (`Ctrl+G`) to print out variable values and debug information.
- Review Results:
- Validate the output at each step of the calculation to ensure intermediate values are correct.
Potential Fixes
- Correct the Formula:
- Address any logical or syntax errors found during the review.
- Data Cleaning:
- If errors are due to unexpected data, implement data validation or cleaning before the calculation.
- Error Handling in Code:
- Use `IsError` function or `If…Then` constructs to handle potential divides by zero or other mathematical errors.
- Loop through Data:
- If calculations involve multiple rows, ensure loops correctly iterate through data without skipping or repeating.
- Modularize Code:
- Break down complex calculations into smaller, manageable functions or subroutines. This can help in isolating issues.
- Review References:
- Confirm that all cell or range references in your VBA code are correct and intended.
- Testing:
- After making changes, conduct thorough testing with different data scenarios to validate the fixes.
Example VBA Function Debugging
Here’s an example illustrating basic debugging in a function:
Function CalculateTotal(ByVal price As Double, ByVal quantity As Integer) As Double
On Error GoTo ErrorHandler
Dim total As Double
' Simple calculation
total = price * quantity
' Print debug information
Debug.Print "Price: " & price
Debug.Print "Quantity: " & quantity
Debug.Print "Total: " & total
' Return calculated total
CalculateTotal = total
Exit Function
ErrorHandler:
MsgBox "Error calculating total: " & Err.Description
CalculateTotal = -1 ' Return a specific number indicating failure
End Function
By following these steps, you can effectively find and fix errors in calculated fields using VBA. Keep iterating through debugging and testing until the errors are resolved.