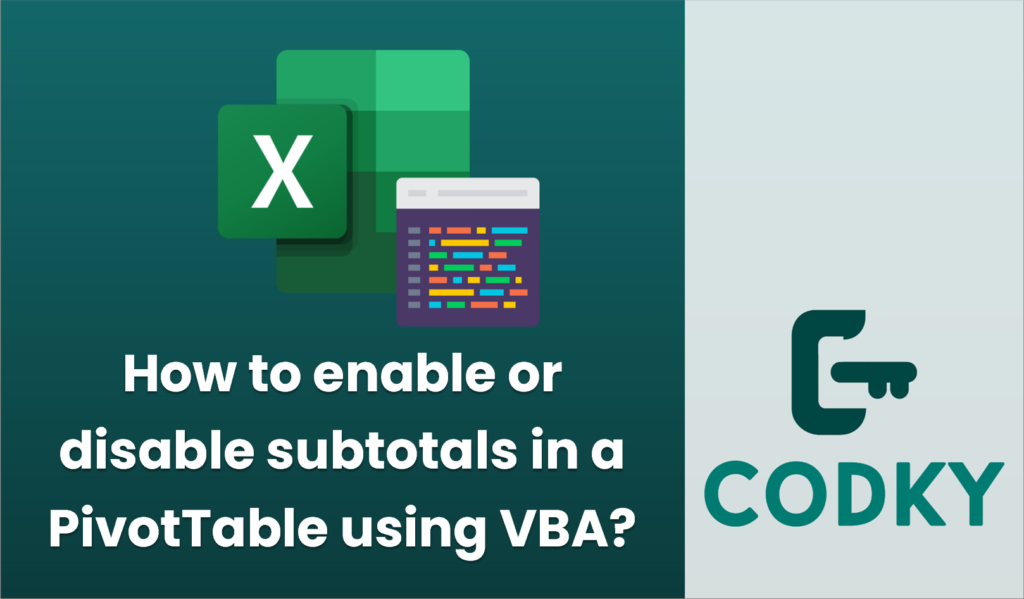
To enable or disable subtotals in a PivotTable using VBA, you need to access the `PivotTable` object and then modify the `Subtotals` property of the `PivotField` objects. Here’s how you can do it:
Enabling Subtotals
To enable subtotals for a specific field, you need to set the `Subtotals` property to an array with `True` for the desired subtotal types. For example, to enable the automatic subtotal (which is typically the Sum), you can do the following:
Sub EnableSubtotals()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
' Reference to your worksheet and PivotTable
Set ws = ThisWorkbook.Worksheets("Sheet1")
Set pt = ws.PivotTables("PivotTable1")
' Reference to the specific PivotField
Set pf = pt.PivotFields("FieldName")
' Enable automatic subtotals
pf.Subtotals(1) = True
End Sub
Disabling Subtotals
To disable subtotals, you set all entries in the `Subtotals` array to `False`.
Sub DisableSubtotals()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
' Reference to your worksheet and PivotTable
Set ws = ThisWorkbook.Worksheets("Sheet1")
Set pt = ws.PivotTables("PivotTable1")
' Reference to the specific PivotField
Set pf = pt.PivotFields("FieldName")
' Disable all subtotals
Dim i As Integer
For i = 1 To 12
pf.Subtotals(i) = False
Next i
End Sub
Explanation:
- Worksheet and PivotTable Reference: Replace `”Sheet1″` with the name of your worksheet and `”PivotTable1″` with the name of your PivotTable.
- PivotField: Replace `”FieldName”` with the name of the field for which you want to change the subtotals setting.
- Enable/Disable Logic:
- `pf.Subtotals(1) = True`: This line enables automatic (typically Sum) subtotals by turning on the first item in the `Subtotals` array.
- The loop that sets `pf.Subtotals(i) = False` sets all 12 possible subtotal types to `False`, effectively disabling any subtotals.
Make sure to replace placeholders with actual names from your Excel workbook to successfully run this script.