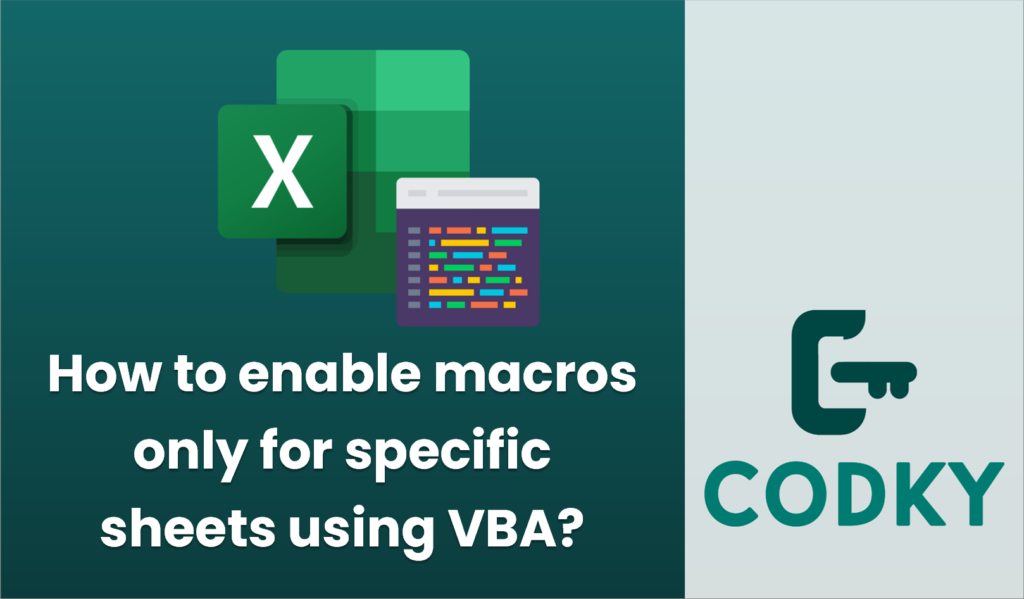
In Excel, VBA (Visual Basic for Applications) macros generally run at the workbook level, affecting all sheets, unless specifically coded otherwise. If you want to enable or run macros only for specific sheets, you need to structure your VBA code such that the macros check which worksheet is active or currently being worked on and only execute operations if certain conditions are met. Here’s a step-by-step guide on how you could achieve this:
Step 1: Open the VBA Editor
- Open your Excel workbook.
- Press `ALT + F11` to open the VBA Editor.
Step 2: Write VBA Code with Sheet-Specific Logic
You can write a macro in a module or directly in the sheets’ code window and specify the condition to run the macro only on certain sheets. Here’s an example of how to do it for a specific sheet:
Example 1: Macro in a Module
To run a macro only for a specific sheet:
Sub RunMacroOnSpecificSheet()
' Define the name of the sheet you want the macro to run on
Dim targetSheetName As String
targetSheetName = "Sheet1" ' Change "Sheet1" to your specific sheet name
' Check if the active sheet name is the target sheet
If ActiveSheet.Name = targetSheetName Then
' Place your macro code here
MsgBox "Running macro on " & targetSheetName
' Example operation: Iterate over cells and perform actions
Dim cell As Range
For Each cell In ActiveSheet.UsedRange
' Perform some action
cell.Value = cell.Value & "_processed" ' Sample operation
Next cell
Else
MsgBox "This macro is not designed to run on this sheet."
End If
End Sub
- Go to `Insert > Module` in the VBA editor to create a new module.
- Insert the following code, adjusting the sheet name and macro logic as needed:
Example 2: Using Worksheet Events
If you want to trigger macros based on worksheet events like activating a sheet:
Private Sub Worksheet_Activate()
' This code runs when a specific sheet is activated
MsgBox "Welcome to " & Me.Name
End Sub
- Select the sheet you want the macro to be associated with in the Project Explorer.
- Double-click the sheet to open its code window.
- Use a worksheet event to determine when to trigger the macro, for example, the `Worksheet_Activate` event:
Step 3: Save Your Workbook
Make sure to save your workbook as a macro-enabled workbook with a `.xlsm` extension.
Step 4: Test Your Macro
- Go back to Excel and run your macro by going to `Developer > Macros` and selecting `RunMacroOnSpecificSheet`, or by activating the appropriate worksheet if using event-based macros.
Important Considerations
- Security Settings: Ensure that your Excel settings allow macros to run. You might need to adjust your macro security settings.
- Error Handling: Incorporate error handling in your VBA code to manage potential runtime errors, particularly when checking sheet names and accessing data.
- Performance: Be mindful of performance considerations when running macros that iterate over large ranges or datasets.
By embedding logic to check and act only on specific sheets, you can control your macro execution scope effectively, ensuring that operations only apply where intended.