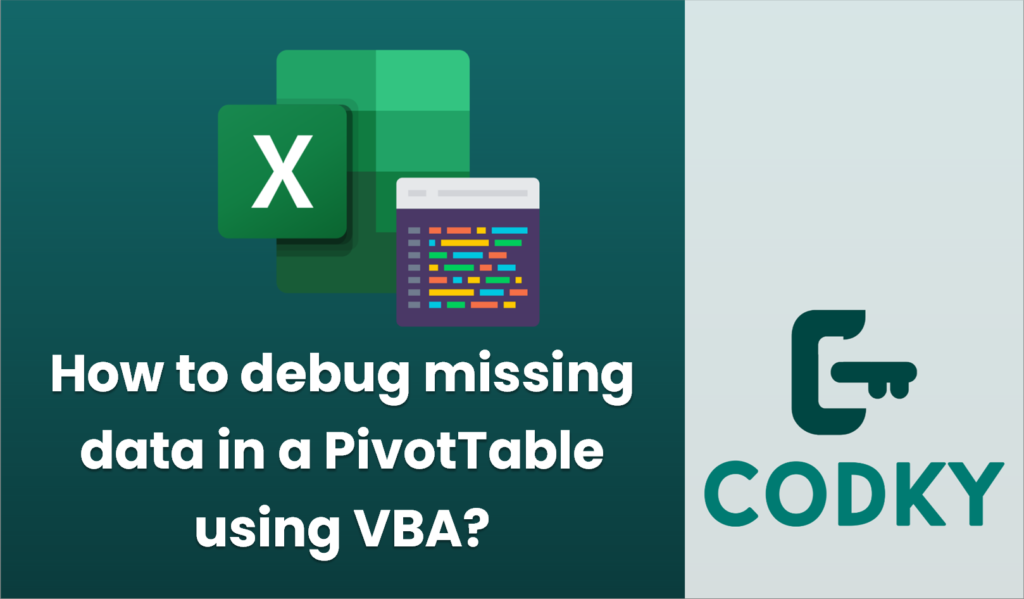
Debugging missing data in a PivotTable using VBA involves identifying potential issues such as incorrect data ranges, filters, or missing source data. Here’s a systematic approach to help diagnose and fix issues:
1. Check the Data Range
Ensure that the PivotTable is based on the correct data range. If rows or columns have been added to your data, make sure the PivotTable source range includes these.
Sub CheckDataRange()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1") ' Adjust as needed
Dim pt As PivotTable
Dim rngAddress As String
For Each pt In ws.PivotTables
rngAddress = pt.SourceData
MsgBox "PivotTable source range is: " & rngAddress
Next pt
End Sub
2. Validate Source Data Completeness
Verify that the source data itself is complete and contains the expected rows and columns.
Sub ValidateSourceData()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("DataSheet") ' Adjust as needed
Dim lastRow As Long
Dim lastCol As Long
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
lastCol = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column
MsgBox "Data Range: A1:" & ws.Cells(lastRow, lastCol).Address
End Sub
3. Check for Filtering
Make sure there are no filters hiding data in the original data set before creating the PivotTable.
Sub CheckFilters()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("DataSheet") ' Adjust as needed
Dim filterRange As Range
Set filterRange = ws.AutoFilter.Range
If ws.AutoFilterMode Then
MsgBox "Filters are active on range: " & filterRange.Address
Else
MsgBox "No filters are currently active."
End If
End Sub
4. Refresh the PivotTable
Often, missing data issues can be resolved by refreshing the PivotTable.
Sub RefreshPivotTable()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1") ' Adjust as needed
Dim pt As PivotTable
For Each pt In ws.PivotTables
pt.RefreshTable
Next pt
End Sub
5. Examine PivotTable Filters and Grouping
Check if the PivotTable itself has filters or groupings that might exclude data.
Sub CheckPivotFilters()
Dim pt As PivotTable
Dim pf As PivotField
Set pt = ThisWorkbook.Sheets("Sheet1").PivotTables("PivotTable1") ' Adjust as needed
For Each pf In pt.PivotFields
If pf.IsMemberProperty Then
' Skip member properties
Else
If pf.VisibleItems.Count < pf.PivotItems.Count Then
MsgBox "Field " & pf.Name & " is filtered."
End If
End If
Next pf
End Sub
6. Update the VBA Code References
Ensure that the VBA code you are using refers to the correct worksheet names, data ranges, and PivotTable names. Double-check these references to avoid errors.
Debugging Steps Recap:
- Confirm the data source range.
- Verify no Excel filters are hiding data.
- Refresh PivotTables to catch any dynamic changes.
- Examine PivotField filters and groupings.
By following these steps, you can systematically identify and resolve issues that cause missing data in a PivotTable. Adjust workbook names, sheet names, and PivotTable names accordingly in the VBA code.