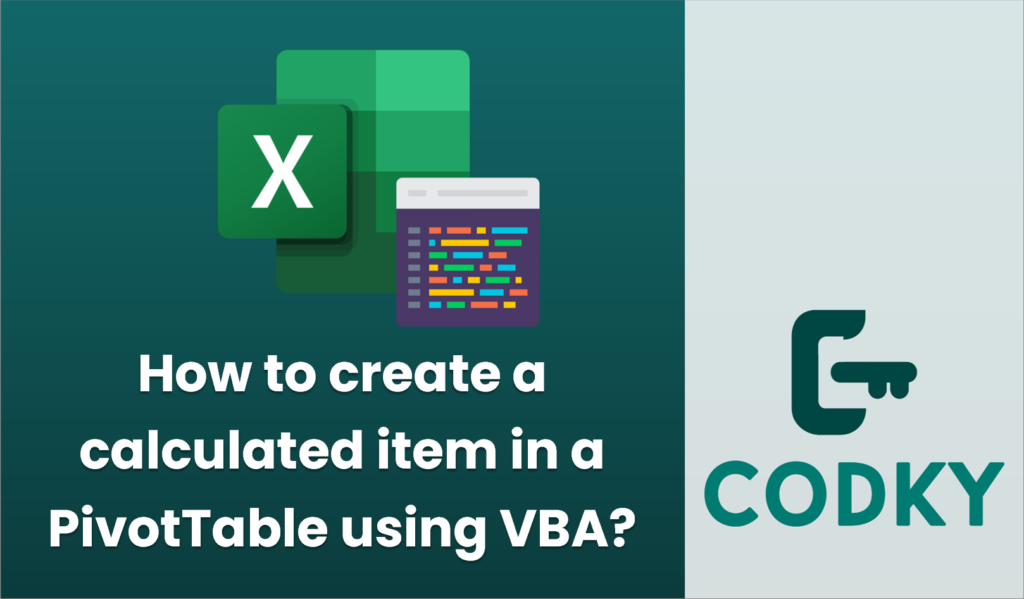
Creating a calculated item in a PivotTable using VBA involves several steps. A calculated item is a custom formula that operates on items within a PivotTable field. Here’s how you can do it:
Step-by-Step Guide
Sub AddCalculatedItem()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
Dim formula As String
' Set the worksheet and PivotTable
Set ws = ThisWorkbook.Worksheets("Sheet1") ' Change to your sheet name
Set pt = ws.PivotTables("PivotTable1") ' Change to your PivotTable name
' Set the PivotField where you want to add the calculated item
Set pf = pt.PivotFields("FieldName") ' Change to your field name
' Define the formula for the calculated item
formula = "=Item1 + Item2" ' Change to your calculation formula
' Check if the pivot field is not a data field and add the calculated item
If pf.Orientation <> xlDataField Then
On Error Resume Next
pf.CalculatedItems.Add Name:="CustomCalcItem", Formula:=formula
On Error GoTo 0
Else
MsgBox "Cannot add calculated item to a data field."
End If
End Sub
- Open VBA Editor:
- Press `Alt` + `F11` to open the Visual Basic for Applications editor.
- Insert a Module:
- In the VBA editor, go to `Insert` > `Module` to create a new module where you will write your code.
- Write the VBA Code:
- Below is an example of how you can add a calculated item to a PivotTable. Make sure to modify it according to your specific requirements (e.g., sheet names, PivotTable names, and field/item names).
Notes:
- Field and Item Names: Ensure that the `FieldName`, `Item1`, `Item2`, and formula are accurately defined as per the existing items within your PivotTable.
- Error Handling: This code includes a basic error handler (`On Error Resume Next`) which allows the macro to continue running even if an error occurs (e.g., item already exists). You might want to enhance this part depending on your needs.
- Run the Macro:
- Make sure the PivotTable is properly set up with the necessary fields and items before you run the macro.
- Press `F5` or run the macro from the VBA editor to execute it.
- Verify the Result:
- Check your PivotTable to ensure that the calculated item appears and that it works as expected.
By following these steps, you can automate the process of adding a calculated item to your PivotTable using VBA. Make sure to customize the field names, PivotTable name, and formula to suit your specific situation.