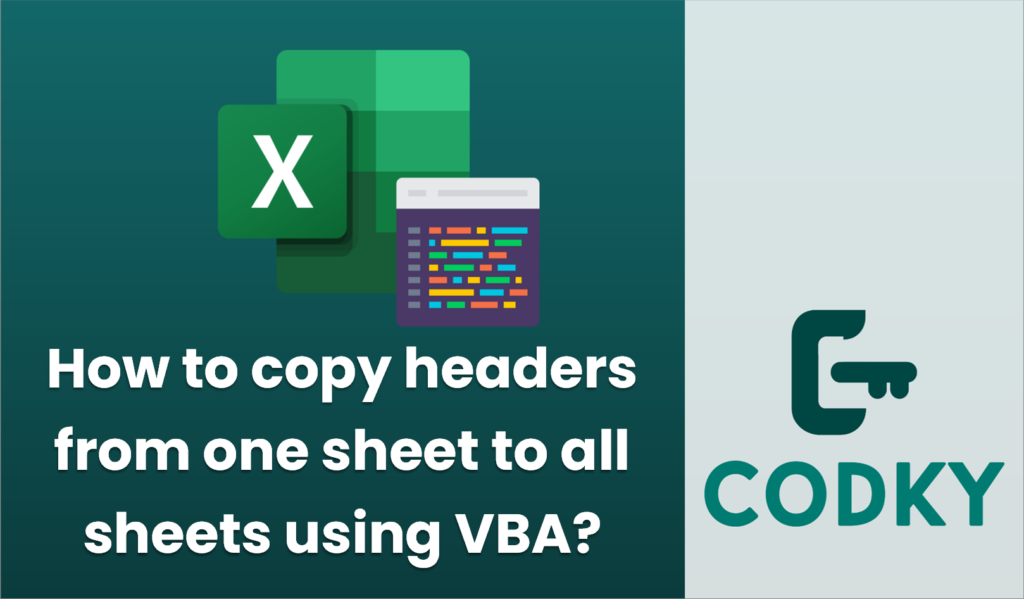
Copying headers from one sheet to all other sheets in an Excel workbook can be accomplished using VBA. Below is a step-by-step guide to create a macro that will achieve this:
Sub CopyHeadersToAllSheets()
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
Dim headerRange As Range
Dim lastCol As Long
Dim sh As Worksheet
' Set the source sheet and header range
Set sourceSheet = ThisWorkbook.Sheets("Sheet1") ' Change "Sheet1" to the name of your header sheet
With sourceSheet
lastCol = .Cells(1, .Columns.Count).End(xlToLeft).Column
Set headerRange = .Range(.Cells(1, 1), .Cells(1, lastCol))
End With
' Loop through all sheets in the workbook
For Each sh In ThisWorkbook.Sheets
' Skip the source sheet
If sh.Name <> sourceSheet.Name Then
With sh
' Copy the header to the target sheet
headerRange.Copy Destination:=.Cells(1, 1)
End With
End If
Next sh
MsgBox "Headers copied to all sheets!"
End Sub
- Open the Visual Basic for Applications Editor:
- Press `ALT + F11` to open the VBA editor in Excel.
- Insert a Module:
- In the VBA editor, go to `Insert` > `Module` to create a new module.
- Write the VBA Code:
- Copy and paste the following code into the module:
- Customize the Code:
- Change `”Sheet1″` to the name of the sheet where your headers are located. Ensure the sheet name is correctly specified, including any spaces or special characters.
- Run the Macro:
- Close the VBA editor.
- Press `ALT + F8` to open the “Macro” dialog.
- Select `CopyHeadersToAllSheets` from the list.
- Click `Run`.
Explanation of the Code:
- sourceSheet: This variable is set to the sheet from which you want to copy the headers.
- headerRange: Determines the range of the header by finding the last used column in the first row.
- Loop Through Sheets: The macro loops through all sheets in the workbook, skipping the one with the headers, and copies the header row to the first row of each target sheet.
Make sure to save your workbook before running the macro, as VBA actions cannot be undone. This way, you can easily retrieve your data if something doesn’t work as intended.