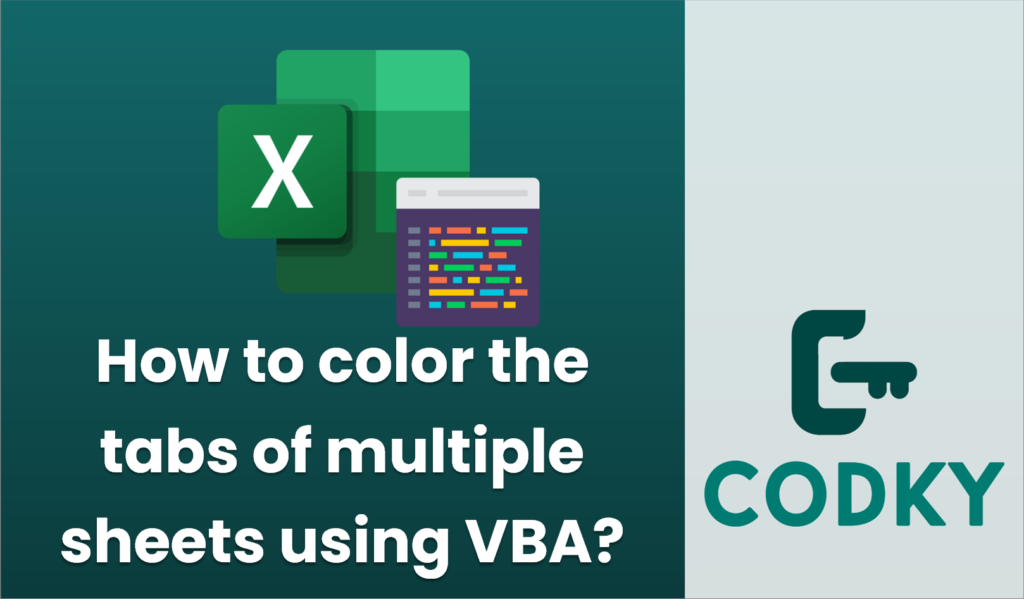
To color the tabs of multiple sheets in Excel using VBA, you can use the `Tab.ColorIndex` property for each worksheet. Below is a simple example of how you can achieve this:
Sub ColorSheetTabs()
Dim ws As Worksheet
' Iterate over each worksheet in the active workbook
For Each ws In ThisWorkbook.Worksheets
' Set the tab color using ColorIndex
' ColorIndex 3 is red, for example. You can change it to other numbers to get different colors
ws.Tab.ColorIndex = 3
Next ws
End Sub
- Open Excel and press `ALT + F11` to open the VBA editor.
- Go to `Insert > Module` to create a new module.
- Copy and paste the following code into the module:
- You can modify the `ColorIndex` value to set different colors for the tabs. Here are some `ColorIndex` values you might use:
- 1: Black
- 2: White
- 3: Red
- 4: Green
- 5: Blue
- 6: Yellow
- Close the VBA Editor and go back to Excel.
- Run your macro by pressing `ALT + F8`, select `ColorSheetTabs`, and click `Run`.
This will change the color of all worksheet tabs in the active workbook to the color specified by the `ColorIndex`. You can adjust the script to choose specific sheets or different colors for each sheet by altering the loop and adding additional logic.
If you prefer to use RGB colors instead of the `ColorIndex`, you can replace `ws.Tab.ColorIndex = 3` with `ws.Tab.Color = RGB(255, 0, 0)` for a custom color. This example will color the tabs red, and you can adjust the RGB values as needed.