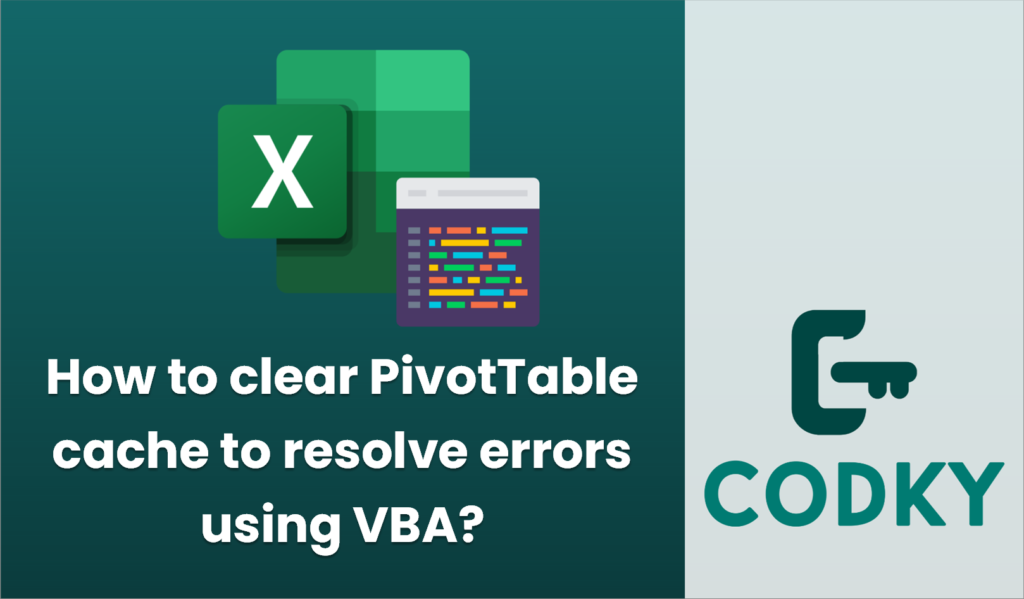
When working with PivotTables in Excel, especially when dealing with updated or changed data sources, you may encounter errors due to outdated cache data. To clear the PivotTable cache using VBA (Visual Basic for Applications), you can write a macro to refresh or clear the data cache associated with your PivotTables. Here’s a step-by-step guide on how to achieve this:
Sub ClearPivotTableCache()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pc As PivotCache
' Iterate through each worksheet in the active workbook
For Each ws In ThisWorkbook.Worksheets
' Iterate through each PivotTable in the worksheet
For Each pt In ws.PivotTables
' Clear the cache
Set pc = pt.PivotCache
pc.MissingItemsLimit = xlMissingItemsNone
pt.RefreshTable
Next pt
Next ws
MsgBox "PivotTable cache cleared and refreshed!"
End Sub
- Open the VBA Editor:
- Press `ALT` + `F11` to open the VBA editor in Excel.
- Insert a New Module:
- In the VBA editor, go to `Insert` > `Module` to create a new module.
- Write the VBA Code:
- Copy and paste the following code into the module. This code iterates through each PivotTable in the active workbook and clears its cache.
- Run the Macro:
- Close the VBA editor or keep it open.
- Press `ALT` + `F8` to bring up the ‘Run Macro’ dialog box.
- Select `ClearPivotTableCache` and click `Run`.
Explanation of the Code:
- Worksheets Loop: The code iterates over each worksheet in the active workbook.
- PivotTables Loop: For every worksheet, it checks all the PivotTables.
- PivotCache: For each PivotTable, it retrieves its PivotCache.
- MissingItemsLimit: `xlMissingItemsNone` is set for the cache to reduce old items that are no longer part of the data source.
- RefreshTable: Refreshes the PivotTable to update the data.
Additional Tips:
- Backup Your Workbook: Always make sure you have a backup of your data before running VBA scripts.
- Adjust Code for Specific Needs: You can modify the code if you want to clear the cache of specific PivotTables by adding conditions to the loops.
By using this VBA method, you can effectively handle cache-related issues in your Excel PivotTables and ensure they are working with the most current data.