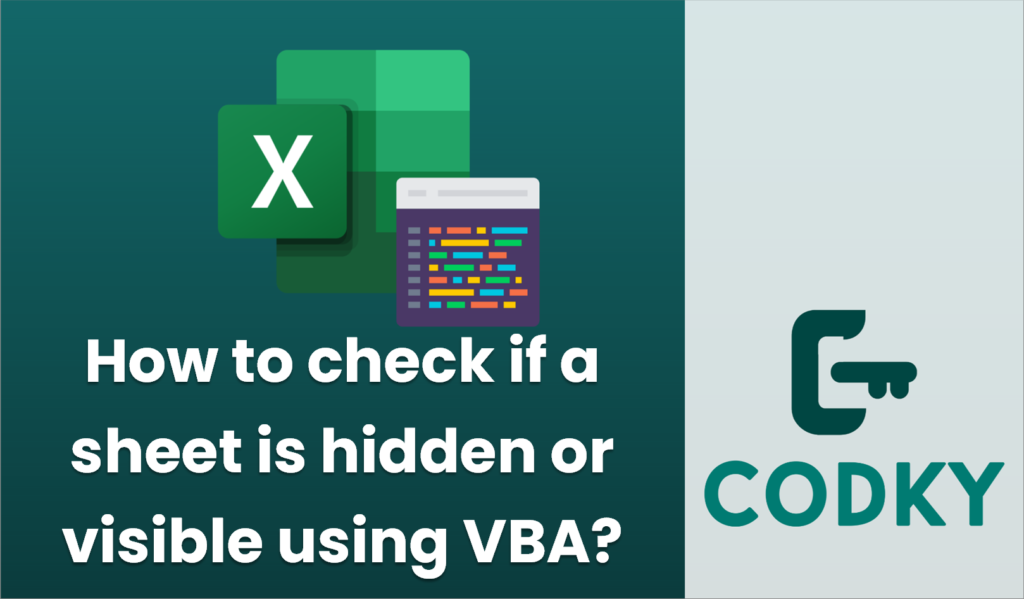
To check if an Excel sheet is hidden or visible using VBA, you can examine the `Visible` property of the worksheet. The `Visible` property can have three possible values:
- `xlSheetVisible` (-1): The sheet is visible.
- `xlSheetHidden` (0): The sheet is hidden but can be unhidden by the user.
- `xlSheetVeryHidden` (2): The sheet is hidden and cannot be unhidden by the user through the Excel interface.
Here’s a simple example of how you can check the visibility status of a specific worksheet by using VBA:
Sub CheckSheetVisibility(sheetName As String)
Dim ws As Worksheet
On Error Resume Next ' In case the sheet does not exist
Set ws = ThisWorkbook.Worksheets(sheetName)
On Error GoTo 0 ' Reset error handling
If ws Is Nothing Then
MsgBox "Sheet does not exist."
Exit Sub
End If
Select Case ws.Visible
Case xlSheetVisible
MsgBox "The sheet is visible."
Case xlSheetHidden
MsgBox "The sheet is hidden."
Case xlSheetVeryHidden
MsgBox "The sheet is very hidden."
Case Else
MsgBox "Unknown visibility state."
End Select
End Sub
To use this subroutine, simply call it from another macro and pass the name of the worksheet you want to check. For example:
Sub ExampleUsage()
Call CheckSheetVisibility("Sheet1")
End Sub
This code will display a message box indicating whether the specified sheet is visible, hidden, or very hidden.