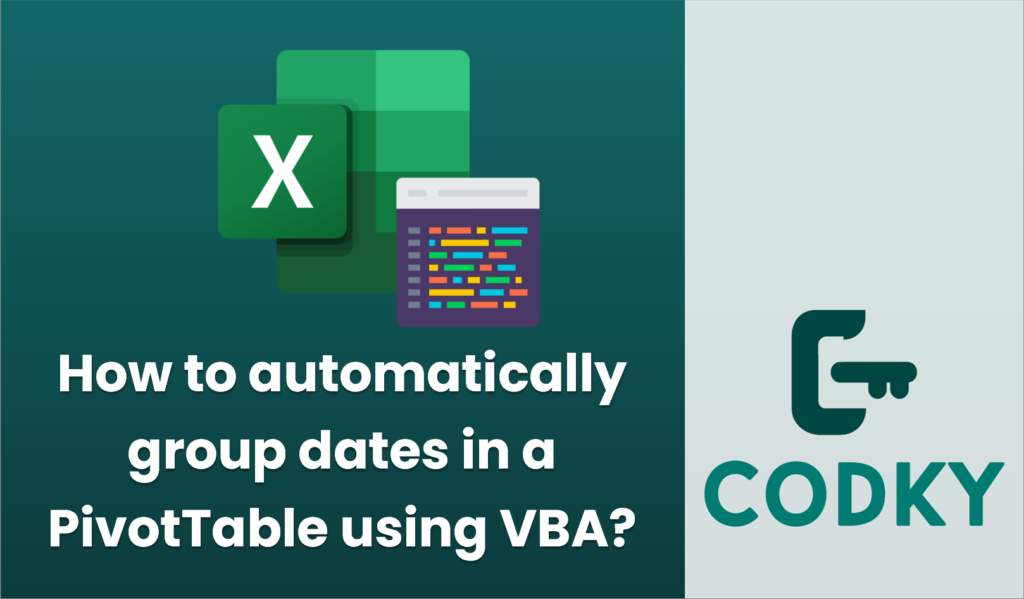
To automatically group dates in a PivotTable using VBA, you can write a macro that follows these steps:
- Create or identify the PivotTable where the dates need to be grouped.
- Access the date field in the PivotTable.
- Use the `PivotField.Group` method to group the dates. You can specify grouping by months, quarters, years, etc.
Here’s an example VBA code snippet that demonstrates how to group dates by months and years in a PivotTable:
Sub GroupDatesInPivotTable()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
' Set the worksheet reference where the PivotTable is located
Set ws = ThisWorkbook.Sheets("Sheet1") ' Change "Sheet1" to your sheet's name
' Set the PivotTable reference
' Assuming there's only one PivotTable in the sheet. Adjust as necessary
Set pt = ws.PivotTables(1)
' Set the PivotField that contains the dates
' Change "Date" to the name of your date field
Set pf = pt.PivotFields("Date")
' Clear any existing grouping
pf.ClearAllFilters
' Group the field by Months and Years
On Error Resume Next ' Suppress errors for already grouped fields
pf.Group Start:=True, End:=True, _
By:=Array(1, 2) ' 1 for Year, 2 for Month; modify array to change grouping
On Error GoTo 0 ' Re-enable error warnings
End Sub
Key Points:
- Worksheet Reference: Ensure that you set the correct worksheet reference (`Sheet1` in this example) where your PivotTable exists.
- PivotTable Reference: The example assumes there’s only one PivotTable in the worksheet. If there are multiple PivotTables, you’ll need to specify the correct one.
- Field Name: Replace `”Date”` with the actual name of your date field in the PivotTable.
- Error Handling: The code contains error handling for cases where the field might already be grouped.
Customization:
- Grouping Levels: Adjust the array in the `Group` method to specify different levels of grouping (e.g., quarters, days).
- PivotTables with Multiple Fields: If your PivotTable has multiple fields, ensure you accurately identify and set the correct `PivotField`.
After setting up your macro, you can run it in Excel’s VBA editor to group dates in your PivotTable according to the specified criteria.