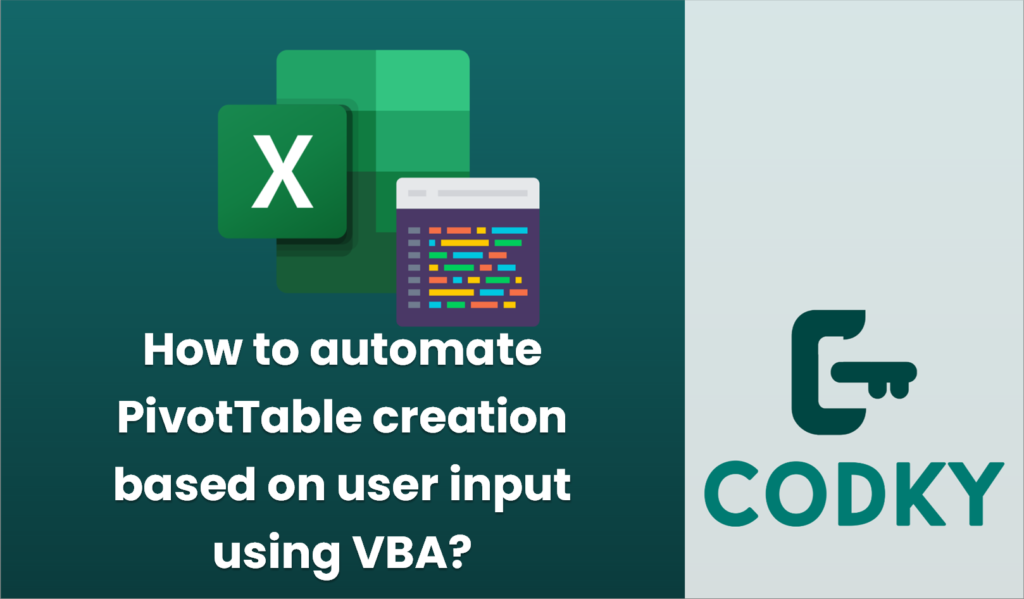
Automating the creation of PivotTables based on user input in Excel using VBA can be accomplished by writing a macro that interacts with the user to collect necessary parameters. Here’s a step-by-step guide to achieve this:
Step 1: Enable the Developer Tab
Make sure the Developer tab is enabled in Excel:
- Go to File > Options.
- Select Customize Ribbon.
- Check the Developer option and click OK.
Step 2: Open the VBA Editor
- Click on the Developer tab.
- Click on Visual Basic to open the VBA Editor.
Step 3: Create a New Module
- In the VBA Editor, click Insert > Module to create a new module.
- This is where you will write your VBA code.
Step 4: Write the VBA Code
Below is a sample code to create a PivotTable based on user input:
Sub CreatePivotTable()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pc As PivotCache
Dim dataRange As Range
Dim userInput As String
Dim pivotRange As String
Dim pivotSheetName As String
' Get user input for data range
userInput = InputBox("Enter the data range (e.g., 'Sheet1!A1:D100'):", "Data Range")
' Parse sheet name and range from user input
pivotSheetName = Split(userInput, "!")(0)
pivotRange = Split(userInput, "!")(1)
' Set the data range
Set dataRange = Evaluate(userInput)
' Create a new worksheet for PivotTable
Set ws = ThisWorkbook.Worksheets.Add
ws.Name = "PivotSheet"
' Create Pivot Cache
Set pc = ThisWorkbook.PivotCaches.Create(SourceType:=xlDatabase, SourceData:=dataRange)
' Create Pivot Table
Set pt = pc.CreatePivotTable(TableDestination:=ws.Cells(1, 1), TableName:="PivotTable1")
' Add fields to the Pivot Table (customize as needed)
' Row Fields
pt.PivotFields("Column1").Orientation = xlRowField
' Column Fields
' pt.PivotFields("Column2").Orientation = xlColumnField
' Data Fields
pt.AddDataField pt.PivotFields("Column3"), "Sum of Column3", xlSum
' Inform the user
MsgBox "PivotTable created on new sheet 'PivotSheet'."
End Sub
Step 5: Run the Macro
- Go back to Excel, click on Macros in the Developer tab.
- Select the `CreatePivotTable` macro and click Run.
- Enter the data range in the prompt (e.g., `Sheet1!A1:D100`).
Important Notes:
- Field Names: Replace `”Column1″`, `”Column2″`, and `”Column3″` with the actual column names from your dataset.
- Destination Sheet: The macro creates a new worksheet for the PivotTable named “PivotSheet”. Modify if needed.
- Data Manipulation: Customize the Row, Column, and Data fields in the code to fit your needs.
This VBA script is a starting point and can be extended with more complexity to handle advanced requirements, such as multiple data fields, different aggregation methods, or more user inputs for customization.