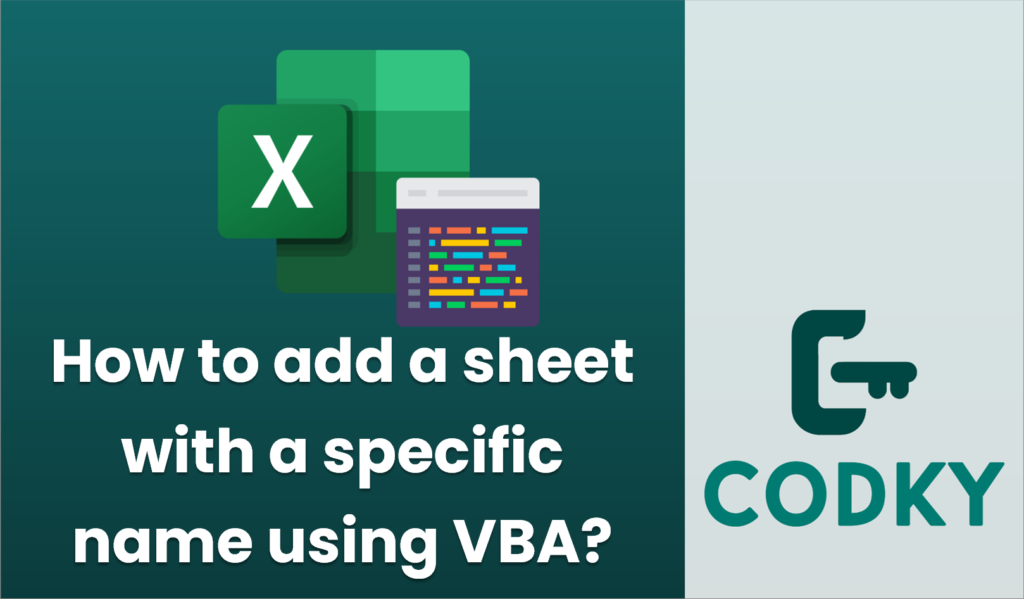
To add a new worksheet with a specific name using VBA in Excel, you can follow the steps below. This will create a macro that adds a new sheet and assigns it the desired name.
Here’s a simple example of how you can do it:
Sub AddSheetWithSpecificName()
Dim sheetName As String
sheetName = "YourSheetName" ' Replace "YourSheetName" with the desired sheet name
' Check if the sheet already exists
On Error Resume Next
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets(sheetName)
On Error GoTo 0
If ws Is Nothing Then
' Add the new sheet
Set ws = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
ws.Name = sheetName
Else
MsgBox "A sheet with the name '" & sheetName & "' already exists.", vbExclamation
End If
End Sub
- Open the VBA Editor:
- Press `ALT` + `F11` in Excel to open the VBA editor.
- Insert a New Module:
- In the VBA editor, go to `Insert` > `Module` to insert a new module.
- Write the VBA Code:
- In the module window, you can write the following code:
- Run the Macro:
- Press `F5` or go to `Run` > `Run Sub/UserForm` to execute the code.
Explanation:
- Variable Declaration: A `sheetName` variable is declared to hold the desired name for the new sheet.
- Checking if the Sheet Already Exists:
- It checks if a sheet with the specified name already exists using `On Error Resume Next`. If it exists, it alerts the user with a message box, preventing errors.
- Adding the Sheet:
- If the sheet doesn’t exist, it adds a new sheet at the end of the current sheets collection and assigns it the specified name.
- Messages and Alerts:
- If a sheet with the desired name already exists, it notifies the user.
Remember to replace `”YourSheetName”` with the name you want for your new worksheet. This script should be run with the workbook where you wish to add the new sheet open and accessible.