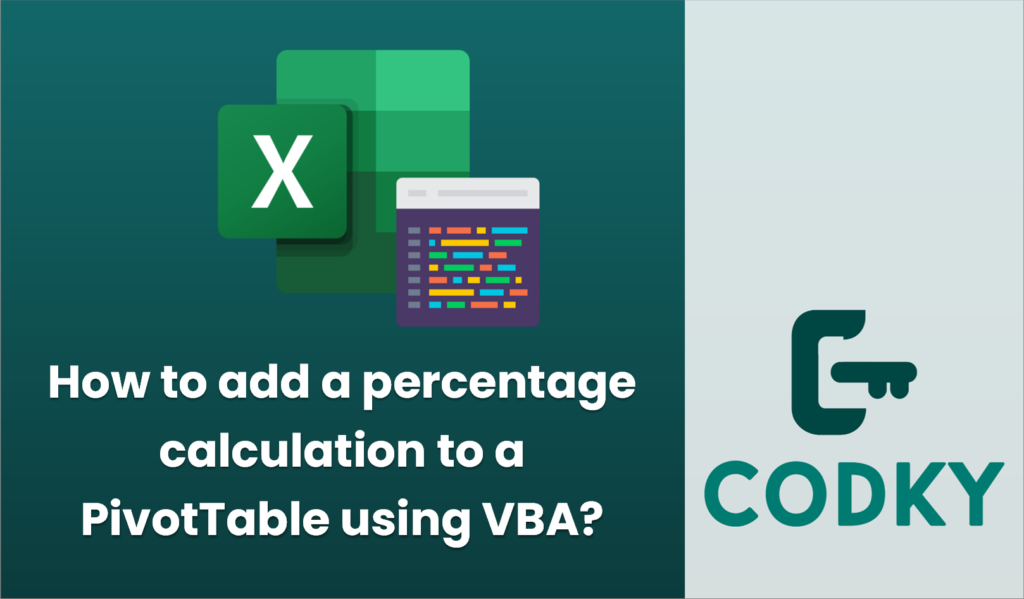
To add a percentage calculation to a PivotTable using VBA, you can follow these general steps. Suppose you already have a PivotTable created, and you want to add a percentage calculation based on certain fields in the table. Here’s a simple example of how you can achieve that using VBA.
Step-by-Step Guide:
Sub AddPercentageToPivotTable()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
Dim totalField As PivotField
Dim dataField As String
Dim totalFieldName As String
Dim calcItem As PivotItem
' Set the worksheet and pivot table
Set ws = ThisWorkbook.Sheets("Sheet1") ' Change to your sheet name
Set pt = ws.PivotTables("PivotTable1") ' Change to your pivot table name
' Specify the field names
dataField = "Sales" ' Change to your data field name
totalFieldName = "Sales % of Total" ' Name for the percentage field
' Add a calculated field to the PivotTable
pt.CalculatedFields.Add Name:=totalFieldName, Formula:="=" & dataField & "/SUM(" & dataField & ")", UseStandardFormula:=True
' Get the reference of the new calculated field
Set pf = pt.PivotFields(totalFieldName)
' Set the number format to percentage
pf.NumberFormat = "0.00%"
' Update the PivotTable
pt.RefreshTable
End Sub
- Open the VBA Editor:
- Press `ALT + F11` in Excel to open the VBA editor.
- Insert a Module:
- Go to `Insert` > `Module` to add a new module where you can write your VBA code.
- Write the VBA Code:
- Below is a sample VBA code that adds a percentage field to an existing PivotTable:
Explanation:
- Worksheet and PivotTable: Identify the worksheet and the PivotTable where you want to add the percentage calculation.
- Field Names: Define the names of the data fields you’re working with. In this example, `dataField` is assumed to be a numeric field like “Sales”.
- Calculated Field: A calculated field is added to the PivotTable using the formula `=field/SUM(field)`, which calculates the percentage of the total for each row.
- Number Format: The number format for the calculated field is set to show as a percentage.
- Refresh: The PivotTable is refreshed to apply changes.
Important Notes:
- Adjust the sheet and PivotTable names to match those in your workbook.
- Ensure that the field names used in the formula are exactly as they appear in your PivotTable.
- Validity of fields and the structure of your PivotTable can affect how a calculated field can be added.
This code snippet should help you add a percentage calculation to a PivotTable programmatically using VBA. Adjust the field names and structure based on your specific PivotTable configuration.