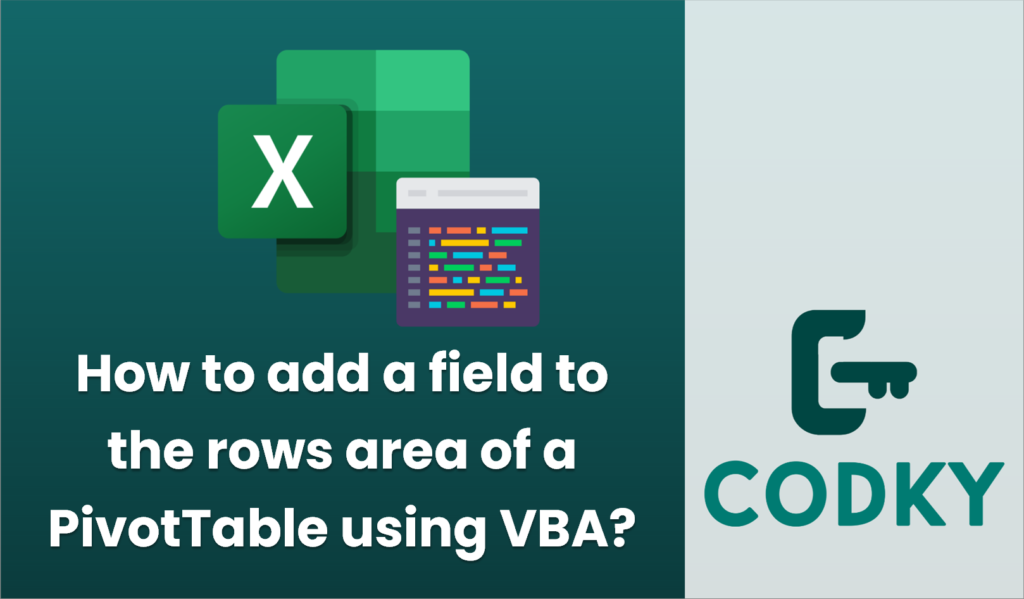
To add a field to the Rows area of a PivotTable using VBA in Excel, you’ll need to access the PivotTable object and modify its PivotFields accordingly. Below is a step-by-step guide and a sample VBA code snippet that demonstrates how to achieve this:
- Access the PivotTable: Identify the worksheet and the PivotTable you want to manipulate.
- Define the PivotField: Specify the field that you want to add to the Rows area.
- Add the Field to the Rows Area: Use the `AddFields` method to add the desired field to the Rows area.
Here is an example of how you can write a VBA macro to add a field to the Rows area of a PivotTable:
Sub AddFieldToRowsArea()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
Dim ptName As String
Dim fieldName As String
' Specify the name of the worksheet containing the PivotTable
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Specify the name of the PivotTable
ptName = "PivotTable1"
' Specify the field name you want to add to the Rows area
fieldName = "YourFieldName"
' Access the PivotTable
Set pt = ws.PivotTables(ptName)
' Find the PivotField
Set pf = pt.PivotFields(fieldName)
' Add the field to the Rows area
With pt
.ManualUpdate = True ' Stops updates until we're done
pf.Orientation = xlRowField
pf.Position = 1 ' Position can be adjusted as needed
.ManualUpdate = False ' Enable updates after we're done
End With
' Optionally, refresh the PivotTable
pt.RefreshTable
End Sub
Explanation:
- ws: Represents the worksheet containing your PivotTable.
- pt: Refers to the PivotTable object.
- pf: Represents the specific PivotField you want to add to the Rows area.
- ManualUpdate: Stops intermediate updates to improve the performance while setting the PivotTable configuration.
- pf.Orientation = xlRowField: Sets the orientation of the field to the Rows area.
- pf.Position = 1: Sets the position of the field in the Rows area. You can adjust the position as needed.
Usage Instructions:
- Open the VBA editor in Excel by pressing `ALT + F11`.
- Insert a new module by going to `Insert > Module`.
- Paste the above code into the module.
- Modify `”Sheet1″`, `”PivotTable1″`, and `”YourFieldName”` to match your worksheet name, PivotTable name, and the field name you want to add.
- Run the macro by placing the cursor inside the Sub and pressing `F5` or by navigating through `Run > Run Sub/UserForm`.
This will add the specified field to the Rows area of the designated PivotTable. Make sure to replace the placeholder names with the actual names used in your workbook.