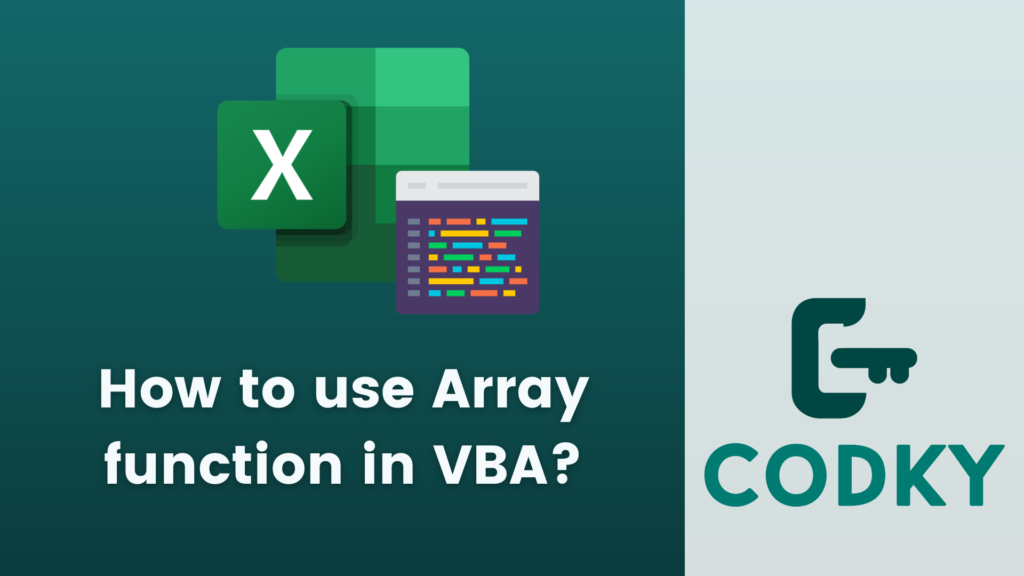
Creating an Array
To create an array, you simply use the Array function and pass the elements you want to include in the array. For example:VBA
In this case, myArray is a variant that holds an array of strings.
Dim myArray As Variant
myArray = Array("Apple", "Banana", "Cherry")
Accessing Elements
You can access elements of the array using their index. Remember that VBA arrays are zero-based by default, so the first element is at index 0.VBA
Dim firstFruit As String
firstFruit = myArray(0) ' This will be "Apple"
Modifying Elements
You can modify elements of the array by accessing them by their index and assigning a new value.VBA
myArray(1) = "Blueberry" ' Changes "Banana" to "Blueberry"
Dynamic Arrays
One of the benefits of using Array function is that it creates a dynamic array. This means you can change the size of the array at runtime.Multidimensional Arrays
While the Array function creates a one-dimensional array, you can nest arrays to create multidimensional arrays.VBA
Dim multiArray As Variant
multiArray = Array(Array(1, 2, 3), Array("A", "B", "C"))
Iterating Over an Array
You can use a loop to iterate over the elements of the array.VBA
Dim item As Variant
For Each item In myArray
Debug.Print item
Next item