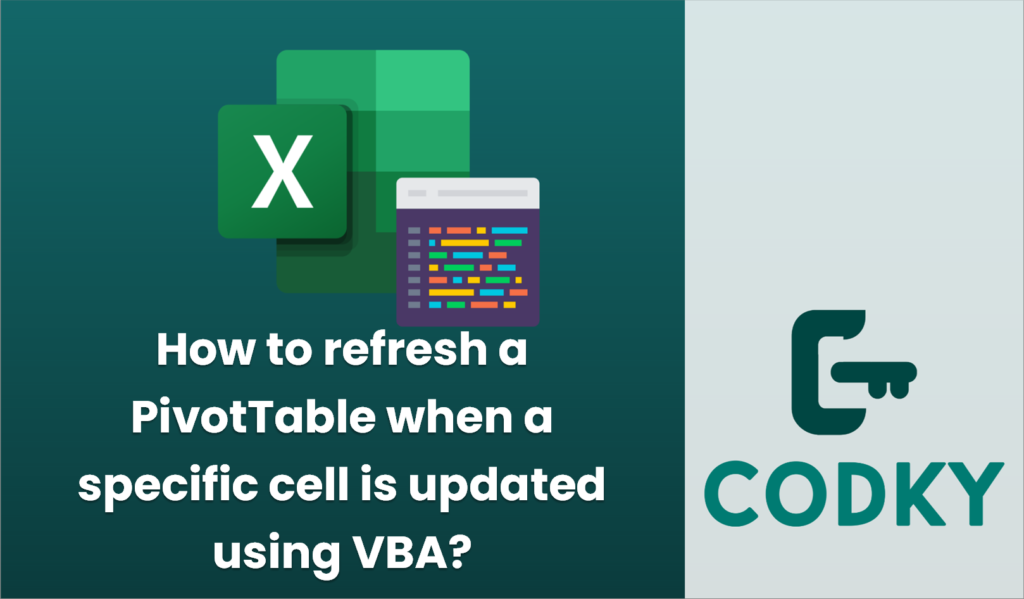
To refresh a PivotTable when a specific cell is updated using VBA, you can use an event-driven macro. Specifically, you’ll want to use the `Worksheet_Change` event to detect changes in the target cell, and then refresh the PivotTable based on that change. Here’s a step-by-step guide on how to do this:
Private Sub Worksheet_Change(ByVal Target As Range)
' Check if the changed cell is the specific cell you want to monitor
If Not Intersect(Target, Me.Range("A1")) Is Nothing Then
' Reference the specific PivotTable to refresh
Dim ws As Worksheet
Set ws = Me ' or specify the worksheet explicitly
Dim pt As PivotTable
Set pt = ws.PivotTables("PivotTable1") ' replace with your PivotTable name
' Refresh the PivotTable
pt.RefreshTable
End If
End Sub
- Press `ALT` + `F11` to open the Visual Basic for Applications (VBA) editor.
- In the Project Explorer window, find the worksheet where you want to monitor the specific cell change (e.g., `Sheet1`).
- Double-click the worksheet name to open the code window for that sheet.
- Enter the following VBA code, replacing `A1` with the address of the cell you want to monitor, and modify the PivotTable parameters as needed:
Explanation:
- `Worksheet_Change`: This is an event that triggers every time a change is made on the worksheet.
- `Target`: Represents the range of cells that were changed.
- `Intersect`: This function checks whether the cell that was changed (`Target`) is the specific cell you want to monitor (e.g., `A1`). If it is, the `If` condition is satisfied.
- `Me.Range(“A1”)`: Replace `”A1″` with the address of the cell you want to monitor.
- `ws.PivotTables(“PivotTable1”)`: Replace `”PivotTable1″` with the name of your specific PivotTable.
- `pt.RefreshTable`: This method refreshes the PivotTable.
After adding this code to the worksheet, every time the specified cell is updated, the macro will automatically refresh the corresponding PivotTable. Make sure the PivotTable name is correct and matches the name in your worksheet.