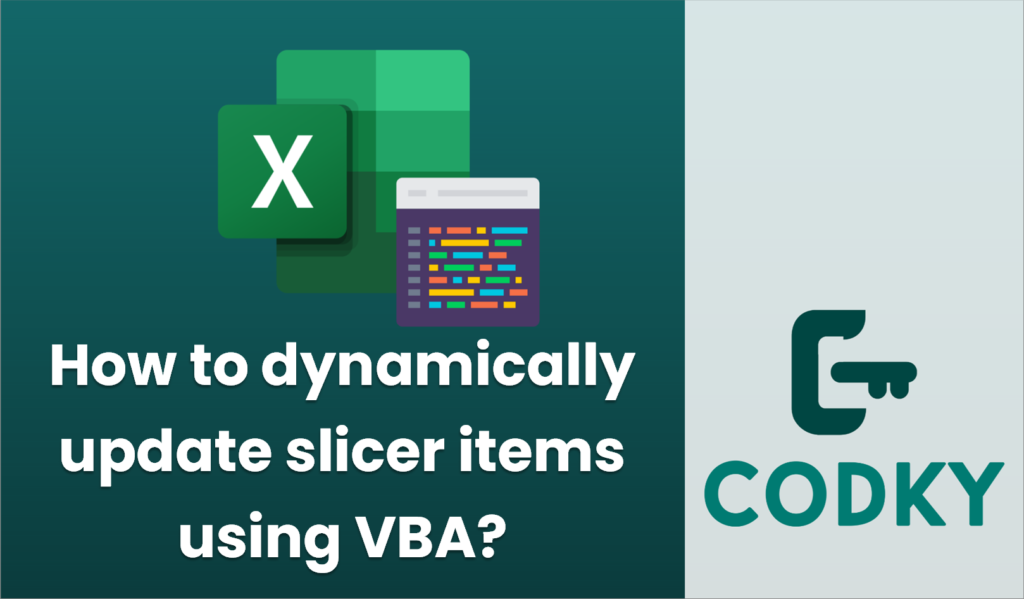
Updating slicer items dynamically using VBA involves a few key steps. You need to ensure that your slicer is connected to a pivot table, and then you can manipulate the slicer items using VBA. Here’s a general approach to achieve this:
Steps to Update Slicer Items Dynamically with VBA:
- Access the Slicer and Pivot Table:
- Ensure your slicer is connected to a pivot table.
- Identify the names of your slicer and pivot table fields.
- Write the VBA Code:
- Open the VBA editor by pressing `ALT + F11`.
- Insert a new module or use an existing one.
- Sample Code for Updating Slicer:
Here is an example code to dynamically update slicer items:
Sub UpdateSlicer()
Dim wb As Workbook
Dim ws As Worksheet
Dim pt As PivotTable
Dim slicerCache As SlicerCache
Dim slicerItem As SlicerItem
' Set workbook and worksheet
Set wb = ThisWorkbook
Set ws = wb.Sheets("Sheet1") ' Specify your sheet name
' Set pivot table
Set pt = ws.PivotTables("PivotTable1") ' Specify your pivot table name
' Set slicer cache
Set slicerCache = wb.SlicerCaches("Slicer_YourField") ' Specify your slicer name
' Loop through each slicer item
For Each slicerItem In slicerCache.SlicerItems
' Update items based on condition
If slicerItem.Name = "SpecificCriteria" Then
slicerItem.Selected = True
Else
slicerItem.Selected = False
End If
Next slicerItem
End Sub
- Customizing the Code:
- Replace `”Sheet1″` with the actual name of your worksheet.
- Replace `”PivotTable1″` with the name of your pivot table.
- Replace `”Slicer_YourField”` with the slicer cache name (this is usually the slicer name prefixed by “Slicer_”).
- Adjust the condition (`If slicerItem.Name = “SpecificCriteria”`) to fit your criteria for selecting slicer items.
- Run the Macro:
- To execute the script, you can run the macro from the VBA editor, or assign it to a button/shortcut in Excel for easier access.
Things to Note:
- Make sure Excel macros are enabled, or the macro won’t run.
- Test your VBA script in a copy of the document to prevent data loss.
- It’s useful to add error handling to manage run-time errors effectively.
By following these steps, your slicer items should update dynamically based on the VBA script conditions. Adjust conditions and objects as needed to fit your specific requirements.