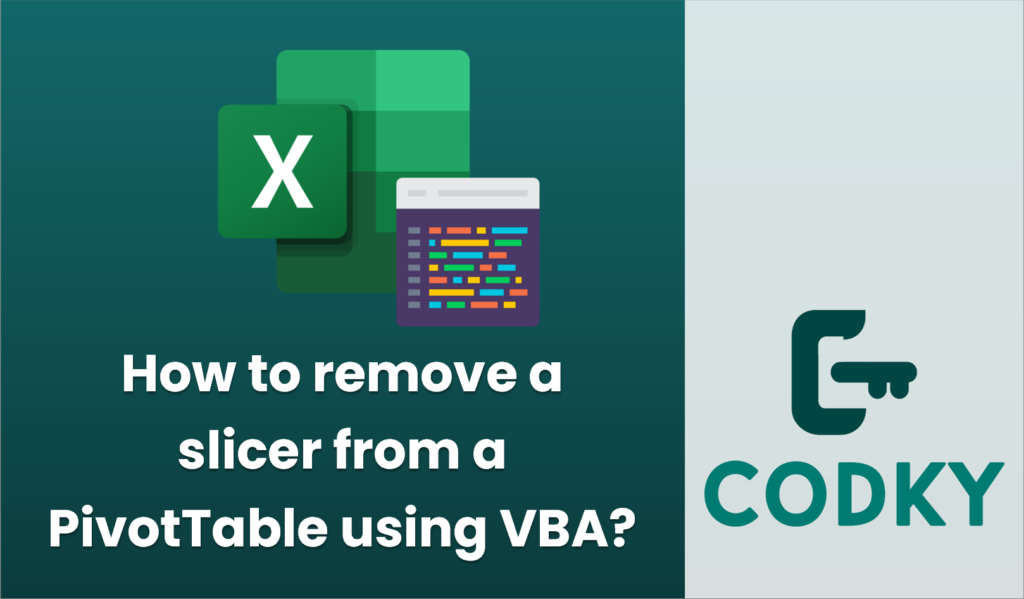
To remove a slicer from a PivotTable using VBA, you need to access the `SlicerCaches` collection, which represents all the slicers in the workbook. Each slicer is represented by a `SlicerCache` object. You can then use the `Delete` method to remove a specific slicer. Here’s a step-by-step guide and example code:
Steps to Remove a Slicer:
- Identify the Slicer: Determine the name of the slicer you want to remove. You can find the slicer name in the Slicer Settings or by selecting the slicer in Excel and checking its properties.
- Access the SlicerCaches: Use the `ThisWorkbook.SlicerCaches` collection to access the slicers.
- Delete the Slicer: Use the `Delete` method on the specific `SlicerCache` you want to remove.
Example VBA Code:
Sub RemovePivotTableSlicer()
Dim ws As Worksheet
Dim slicerName As String
Dim slicerCache As SlicerCache
' Set your specific worksheet that contains the slicer
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Specify the name of the slicer you want to remove
slicerName = "Slicer_ProductCategory"
' Loop through each SlicerCache in the workbook
For Each slicerCache In ThisWorkbook.SlicerCaches
' Check if SlicerCache name matches the specified slicer name
If slicerCache.Name = slicerName Then
' Delete the slicer
slicerCache.Delete
Exit For
End If
Next slicerCache
MsgBox "Slicer removed successfully!"
End Sub
Notes:
- Ensure the slicer name is correct: Double-check the name of the slicer you want to delete. It must match exactly, including any spaces and case sensitivity.
- Remove carefully: This operation cannot be undone, so make sure you really want to remove the slicer.
- Test in a copy: It’s a good practice to test your VBA code on a copy of your data to ensure it behaves as expected.
By using this code, you can efficiently remove a slicer from a PivotTable in Excel using VBA.