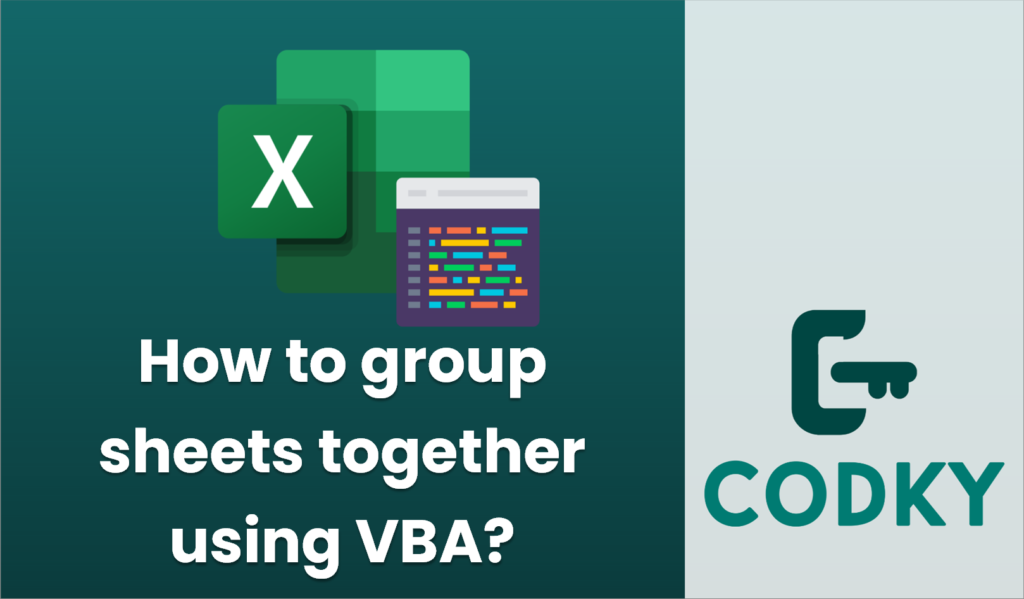
To group sheets together using VBA in Excel, you can use the `Worksheets` or `Sheets` collection to select multiple sheets at once. Grouping sheets allows you to perform operations on all the selected sheets simultaneously. Here’s a step-by-step guide on how you can achieve this:
Sub GroupSheets()
Dim sheetNames As Variant
Dim ws As Worksheet
Dim i As Integer
' Specify the sheet names you want to group
sheetNames = Array("Sheet1", "Sheet2", "Sheet3")
' Loop through and select sheets for grouping
For i = LBound(sheetNames) To UBound(sheetNames)
Set ws = Worksheets(sheetNames(i))
If i = LBound(sheetNames) Then
ws.Select False ' Select the first sheet without extending selection
Else
ws.Select True ' Add remaining sheets to the selection
End If
Next i
End Sub
- Open the Visual Basic for Applications (VBA) Editor:
- Press `ALT` + `F11` in Excel to open the VBA editor.
- Insert a Module:
- Right-click on any of the objects for your workbook in the Project Explorer pane.
- Click on “Insert” and then select “Module” to create a new module.
- Write VBA Code to Group Sheets:
- Write a macro in the module to group sheets. Here is an example code snippet that groups specific sheets by their names:
- Run the Macro:
- Press `F5` while within the VBA editor or go back to Excel, and run the macro by going to the “Developer” tab, clicking on “Macros,” selecting `GroupSheets`, and clicking “Run.”
Explanation:
- The `sheetNames` array contains the names of the sheets that you want to group.
- The loop iterates over each sheet name, selecting the first sheet normally and extending the selection with `.Select True` for the others.
Additional Notes:
- You can adjust the `sheetNames` array to include any sheets you want to group.
- This macro does not work if the sheets you want to group include the active sheet, so ensure the active sheet is not one of those being grouped, or modify the code accordingly.
By using this method, you can group sheets programmatically to perform collective actions such as formatting, data entry, or analysis.