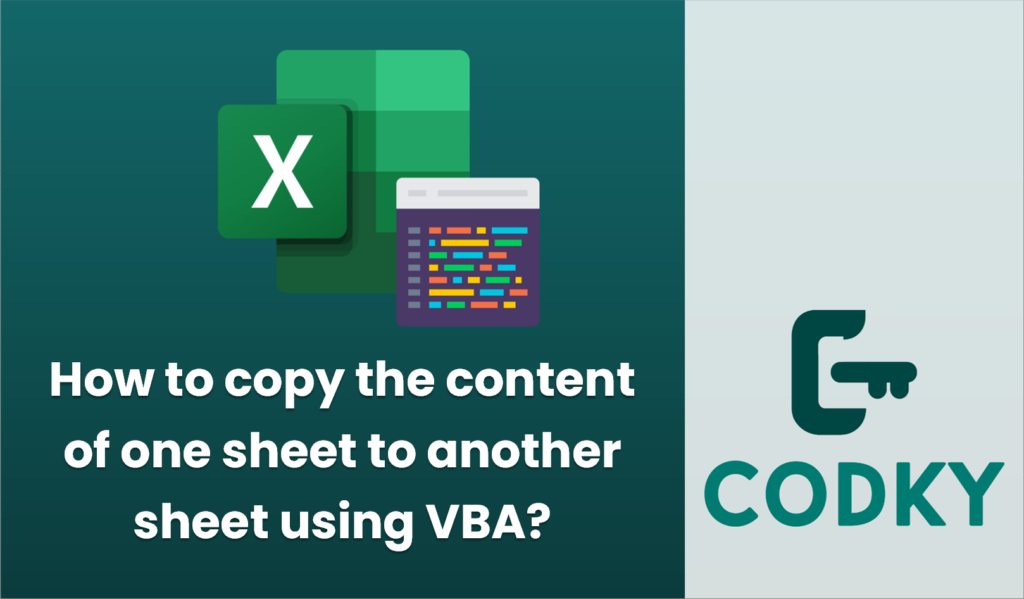
To copy the content of one worksheet to another using VBA in Excel, you can utilize the `Copy` method. Here’s a step-by-step guide on how you can achieve this:
Sub CopySheetContents()
' Define the source and destination worksheets
Dim sourceSheet As Worksheet
Dim destinationSheet As Worksheet
' Set the source and destination worksheets
' Change "Sheet1" and "Sheet2" to your actual sheet names
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set destinationSheet = ThisWorkbook.Sheets("Sheet2")
' Clear the destination sheet before copying
destinationSheet.Cells.Clear
' Copy the entire content from the source sheet
sourceSheet.UsedRange.Copy Destination:=destinationSheet.Range("A1")
' Optionally, you can adjust the destination cell if needed
' For instance, if you want to offset it to start at another cell
'sourceSheet.UsedRange.Copy Destination:=destinationSheet.Range("B1")
' Show a message box to indicate completion
MsgBox "Contents copied from " & sourceSheet.Name & " to " & destinationSheet.Name
End Sub
- Open Excel and Access the Developer Tab:
- Make sure the Developer tab is visible. If not, you can enable it via Excel Options.
- Go to the “Developer” tab and click on “Visual Basic” to open the VBA editor.
- Create a New Module:
- In the VBA editor, right-click on any of the items under “VBAProject” (usually your workbook’s name) and choose “Insert” > “Module”.
- This will create a new module where you will write your code.
- Write the VBA Code:
- In the module window, enter the following code, which will copy the contents of one sheet to another:
- Run the Macro:
- Press `F5` to run the macro, or go back to Excel, click on “Macros” in the Developer tab, select “CopySheetContents”, and click “Run”.
- Customization:
- Replace `”Sheet1″` and `”Sheet2″` with the actual names of your source and destination sheets.
- Modify the destination range if you want the content pasted at a different location other than `A1`.
This code clears the destination sheet before copying the data to prevent any previous content from interfering, and it uses the `UsedRange` property, which ensures that only the filled cells from the source sheet are copied over.