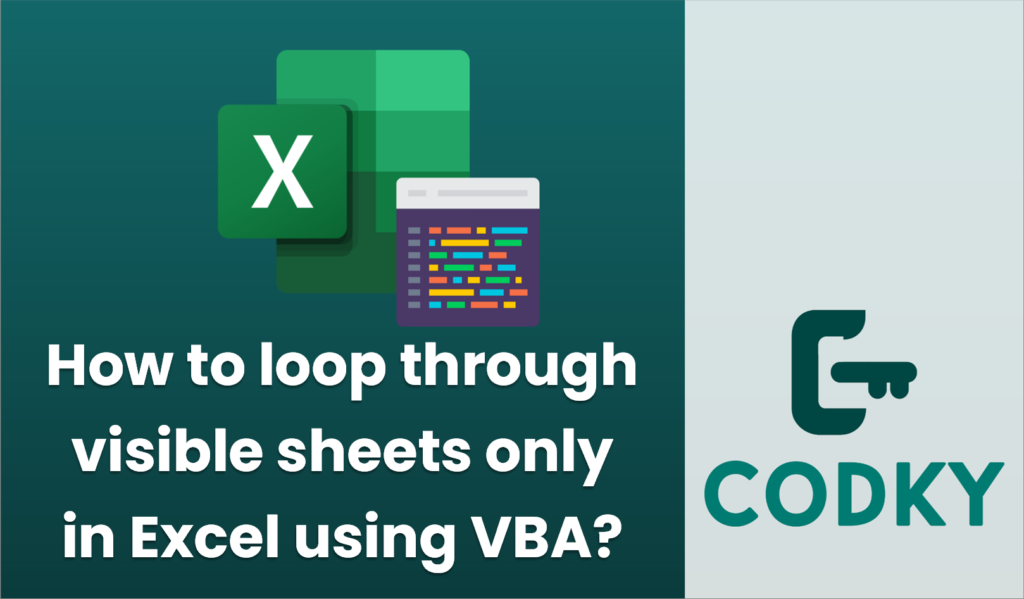
To loop through only the visible sheets in an Excel workbook using VBA, you can use the `Worksheets` collection along with a condition to check the `Visible` property of each sheet. Sheets can have one of three visibility states, represented by the constants `xlSheetVisible`, `xlSheetHidden`, and `xlSheetVeryHidden`.
Here’s a basic example of how you can achieve this:
Sub LoopThroughVisibleSheets()
Dim ws As Worksheet
' Loop through each worksheet in the workbook
For Each ws In ThisWorkbook.Worksheets
' Check if the worksheet is visible
If ws.Visible = xlSheetVisible Then
' Perform your operations here on the visible sheet
' For example, you could display the name of the sheet
Debug.Print ws.Name
End If
Next ws
End Sub
Explanation:
- `ThisWorkbook.Worksheets`: Refers to all the worksheets in the workbook where the code resides. If you need to reference another specific workbook, replace `ThisWorkbook` with that workbook object.
- `ws.Visible = xlSheetVisible`: Ensures the code block inside the `If` statement only executes if the sheet is visible.
- `Debug.Print ws.Name`: This line is just an example action that prints the name of each visible sheet to the Immediate window. You can replace this with any actions you need to perform on each visible sheet.
Remember, this code should be placed in a VBA module. To access the VBA environment, press `ALT + F11` in Excel, then insert a new module and paste the above code there. You can run this macro manually or call it from other procedures as needed.